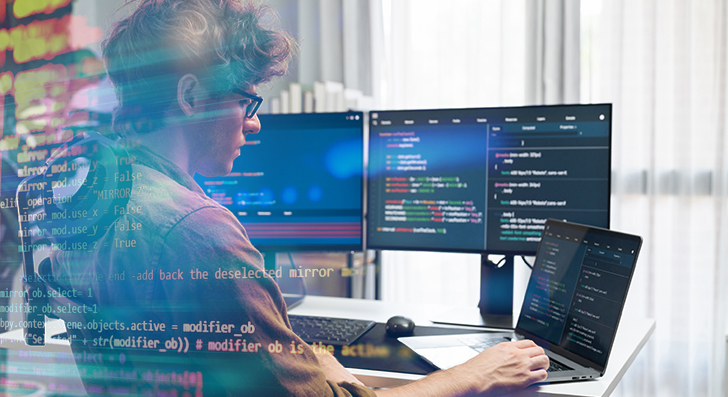
Scalability suggests your application can handle expansion—far more consumers, more details, plus much more targeted traffic—with no breaking. As being a developer, building with scalability in mind will save time and tension afterwards. Listed here’s a transparent and functional manual to help you begin by Gustavo Woltmann.
Design and style for Scalability from the Start
Scalability is not some thing you bolt on later—it ought to be element of your approach from the beginning. Several applications are unsuccessful after they increase quick since the original layout can’t tackle the extra load. For a developer, you might want to Assume early about how your process will behave under pressure.
Start off by designing your architecture for being flexible. Stay clear of monolithic codebases exactly where all the things is tightly connected. As a substitute, use modular design or microservices. These designs crack your application into scaled-down, unbiased elements. Just about every module or services can scale on its own devoid of influencing the whole technique.
Also, contemplate your database from working day a person. Will it need to deal with 1,000,000 buyers or just a hundred? Select the ideal sort—relational or NoSQL—dependant on how your data will develop. Prepare for sharding, indexing, and backups early, Even when you don’t have to have them yet.
Yet another critical place is to avoid hardcoding assumptions. Don’t create code that only performs underneath current situations. Think of what would happen if your user foundation doubled tomorrow. Would your application crash? Would the database slow down?
Use style patterns that help scaling, like concept queues or function-driven techniques. These aid your application cope with extra requests without getting overloaded.
When you build with scalability in your mind, you are not just planning for achievement—you happen to be minimizing foreseeable future head aches. A nicely-prepared procedure is simpler to keep up, adapt, and develop. It’s much better to organize early than to rebuild later.
Use the ideal Databases
Selecting the correct databases is often a essential Portion of developing scalable purposes. Not all databases are built the exact same, and using the wrong you can slow you down or even bring about failures as your app grows.
Start by knowledge your info. Could it be highly structured, like rows in a very table? If Certainly, a relational database like PostgreSQL or MySQL is a good healthy. These are generally strong with associations, transactions, and consistency. In addition they assist scaling techniques like examine replicas, indexing, and partitioning to handle additional site visitors and details.
Should your details is much more adaptable—like consumer exercise logs, solution catalogs, or files—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at dealing with large volumes of unstructured or semi-structured knowledge and can scale horizontally far more very easily.
Also, think about your read through and write patterns. Will you be performing numerous reads with much less writes? Use caching and read replicas. Do you think you're managing a large generate load? Consider databases that will cope with high compose throughput, or maybe event-primarily based info storage devices like Apache Kafka (for short-term knowledge streams).
It’s also good to think forward. You might not will need advanced scaling attributes now, but selecting a database that supports them suggests you received’t require to change later on.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your details based upon your obtain patterns. And always keep track of database functionality while you increase.
In a nutshell, the appropriate databases is dependent upon your application’s construction, pace requirements, And the way you anticipate it to increase. Just take time to choose properly—it’ll conserve a lot of trouble afterwards.
Improve Code and Queries
Rapidly code is vital to scalability. As your app grows, each tiny delay adds up. Improperly published code or unoptimized queries can decelerate efficiency and overload your method. That’s why it’s crucial to build economical logic from the start.
Begin by writing clean, very simple code. Prevent repeating logic and remove something avoidable. Don’t select the most sophisticated solution if a straightforward a single functions. Keep the features quick, focused, and simple to test. Use profiling applications to seek out bottlenecks—places where by your code will take too very long to run or takes advantage of excessive memory.
Up coming, look at your database queries. These often sluggish issues down much more than the code by itself. Make certain Each individual query only asks for the info you really have to have. Avoid Pick out *, which fetches every little thing, and in its place decide on specific fields. Use indexes to hurry up lookups. And steer clear of executing too many joins, Specially throughout huge tables.
For those who detect the same info remaining requested over and over, use caching. Retail outlet the results temporarily employing applications like Redis or Memcached which means you don’t should repeat expensive functions.
Also, batch your databases operations once you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and helps make your application extra efficient.
Remember to check with massive datasets. Code and queries that work high-quality with a hundred documents might crash whenever they have to take care of one million.
In short, scalable apps are rapidly applications. Keep the code tight, your queries lean, and use caching when necessary. These techniques assist your application remain easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your application grows, it's to manage far more people plus much more targeted visitors. If all the things goes as a result of one server, it is going to promptly turn into a bottleneck. That’s the place load balancing and caching are available in. These two resources aid keep your app fast, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout many servers. In place of one server accomplishing many of the get the job done, the load balancer routes people to unique servers based upon availability. What this means is no single server gets overloaded. If one server goes down, the load balancer can send visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing facts briefly so it may be reused quickly. When users ask for the identical info all over again—like a product web page or a profile—you don’t should fetch it within the read more database every time. You could serve it within the cache.
There are 2 common sorts of caching:
1. Server-facet caching (like Redis or Memcached) retailers data in memory for rapidly access.
two. Client-facet caching (like browser caching or CDN caching) merchants static files near to the person.
Caching decreases databases load, enhances velocity, and helps make your application much more successful.
Use caching for things that don’t adjust often. And constantly make sure your cache is current when info does transform.
In brief, load balancing and caching are uncomplicated but powerful equipment. Together, they help your application deal with far more buyers, remain rapidly, and Get better from issues. If you intend to develop, you may need both of those.
Use Cloud and Container Resources
To create scalable purposes, you need resources that allow your application mature easily. That’s in which cloud platforms and containers can be found in. They provide you adaptability, reduce setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon Website Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you require them. You don’t really have to buy hardware or guess long term capacity. When site visitors will increase, it is possible to incorporate additional methods with just some clicks or automatically using auto-scaling. When traffic drops, you are able to scale down to save money.
These platforms also provide solutions like managed databases, storage, load balancing, and security tools. You can focus on setting up your application in place of controlling infrastructure.
Containers are One more crucial Instrument. A container deals your app and everything it really should operate—code, libraries, options—into 1 unit. This can make it effortless to move your app involving environments, from the laptop to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
When your application works by using several containers, resources like Kubernetes assist you take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the app crashes, it restarts it mechanically.
Containers also allow it to be straightforward to individual parts of your application into providers. You can update or scale areas independently, that is perfect for efficiency and reliability.
To put it briefly, making use of cloud and container tools signifies you can scale rapid, deploy effortlessly, and Get well rapidly when challenges occur. In order for you your app to increase without limitations, get started making use of these instruments early. They save time, lessen danger, and make it easier to stay focused on making, not correcting.
Keep track of Anything
If you don’t check your software, you received’t know when things go Improper. Checking allows you see how your app is executing, place challenges early, and make much better choices as your app grows. It’s a key A part of creating scalable devices.
Start by tracking fundamental metrics like CPU utilization, memory, disk Room, and reaction time. These inform you how your servers and products and services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you acquire and visualize this facts.
Don’t just watch your servers—observe your application too. Keep an eye on how long it takes for customers to load web pages, how often problems come about, and the place they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for critical troubles. By way of example, When your response time goes over a limit or a service goes down, you should get notified straight away. This allows you deal with difficulties rapidly, generally ahead of end users even recognize.
Monitoring is usually handy if you make adjustments. In the event you deploy a new aspect and find out a spike in mistakes or slowdowns, you can roll it again just before it leads to serious problems.
As your app grows, traffic and facts boost. Without checking, you’ll skip indications of problems until finally it’s as well late. But with the ideal equipment set up, you keep on top of things.
In a nutshell, monitoring can help you keep your application dependable and scalable. It’s not pretty much spotting failures—it’s about knowledge your program and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t just for significant firms. Even compact apps will need a powerful Basis. By developing diligently, optimizing properly, and utilizing the right equipment, you can Construct applications that develop efficiently without breaking under pressure. Start out small, Feel significant, and Develop sensible.